Input Strings
Programs commonly need to read input typed by the user on the keyboard. We will use the Python functions to do this.
Python's built-in input function to read input from the keyboard. The input
function reads a piece of data that has been entered at the keyboard and
returns that data, as a string, back to the program. You normally use the
input function in an assignment statement that follows this general format:
variable = input(prompt)
Example
Input Numbers
The input function always returns the users input as a string, even if the user enters numeric data. For example, suppose you call the input function, type the number 87, and press the Enter key. The value that is returned from the input function is the string '87'. This can be a problem if you want to use the value in a math operation. Math operations can be performed only on numeric values, not strings.
Function | Description |
---|---|
int(item) | You pass an argument to the int() function and it returns the argument's value converted to an int. |
float(item) | You pass an argument to the float() function and it returns the argument's value converted to a float. |
Method 1
- string_value = input('How many hours did you work? ')
- hours = int(string_value)
Method 2
- hours = int(input('How many hours did you work? '))
Read Multiple Values
Read Multiple Values From keyboard
Comments
Comments are notes of explanation that document lines or sections of a program. Comments are part of the program, but the Python interpreter ignores them. They are intended for people who may be reading the source code.
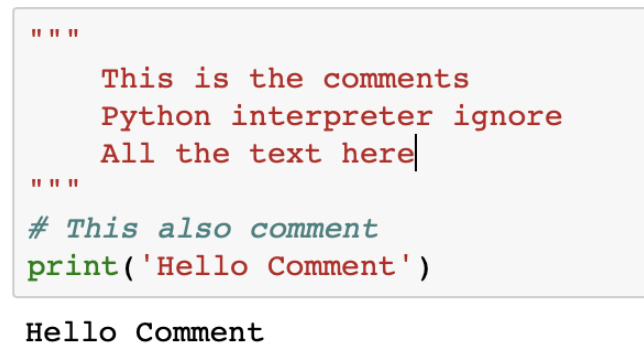